Ever Given¶
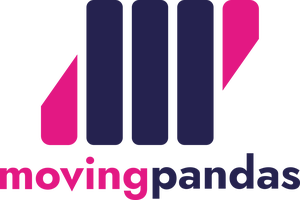
This notebook presents an analysis of the vessel situation following the grounding of Ever Given in the Suez Canal.
The dataset used covers the time span between 2021-03-20 00:00 and 2021-03-24 12:52 UTC.
This data has generously been provided by VesselsValue.

In [1]:
import pandas as pd
import geopandas as gpd
import movingpandas as mpd
from shapely.geometry import Point
from datetime import datetime, timedelta
from hvplot import pandas
from holoviews import opts, dim
from holoviews.selection import link_selections
import warnings
warnings.simplefilter("ignore")
In [2]:
EVERID = 235
FSIZE = 300
In [3]:
df = pd.read_csv("../data/boat-positions.csv")
df["t"] = pd.to_datetime(df["ais_pos_timestamp"], format="%d/%m/%Y %H:%M")
df
Out[3]:
ID | ais_pos_timestamp | longitude | latitude | t | |
---|---|---|---|---|---|
0 | 1 | 20/03/2021 00:22 | 32.32925 | 31.43860 | 2021-03-20 00:22:00 |
1 | 1 | 20/03/2021 01:25 | 32.39860 | 31.40955 | 2021-03-20 01:25:00 |
2 | 1 | 20/03/2021 02:07 | 32.37395 | 31.32413 | 2021-03-20 02:07:00 |
3 | 1 | 20/03/2021 02:33 | 32.35152 | 31.24716 | 2021-03-20 02:33:00 |
4 | 1 | 20/03/2021 02:53 | 32.33933 | 31.20590 | 2021-03-20 02:53:00 |
... | ... | ... | ... | ... | ... |
22282 | 256 | 24/03/2021 11:12 | 32.32908 | 31.19488 | 2021-03-24 11:12:00 |
22283 | 256 | 24/03/2021 11:33 | 32.32909 | 31.19486 | 2021-03-24 11:33:00 |
22284 | 256 | 24/03/2021 11:54 | 32.32910 | 31.19484 | 2021-03-24 11:54:00 |
22285 | 256 | 24/03/2021 12:14 | 32.32908 | 31.19485 | 2021-03-24 12:14:00 |
22286 | 256 | 24/03/2021 12:35 | 32.32909 | 31.19483 | 2021-03-24 12:35:00 |
22287 rows × 5 columns
In [4]:
gdf = gpd.GeoDataFrame(
df.drop(["longitude", "latitude", "ais_pos_timestamp"], axis=1),
crs="epsg:4326",
geometry=[Point(xy) for xy in zip(df.longitude, df.latitude)],
)
gdf
Out[4]:
ID | t | geometry | |
---|---|---|---|
0 | 1 | 2021-03-20 00:22:00 | POINT (32.32925 31.4386) |
1 | 1 | 2021-03-20 01:25:00 | POINT (32.3986 31.40955) |
2 | 1 | 2021-03-20 02:07:00 | POINT (32.37395 31.32413) |
3 | 1 | 2021-03-20 02:33:00 | POINT (32.35152 31.24716) |
4 | 1 | 2021-03-20 02:53:00 | POINT (32.33933 31.2059) |
... | ... | ... | ... |
22282 | 256 | 2021-03-24 11:12:00 | POINT (32.32908 31.19488) |
22283 | 256 | 2021-03-24 11:33:00 | POINT (32.32909 31.19486) |
22284 | 256 | 2021-03-24 11:54:00 | POINT (32.3291 31.19484) |
22285 | 256 | 2021-03-24 12:14:00 | POINT (32.32908 31.19485) |
22286 | 256 | 2021-03-24 12:35:00 | POINT (32.32909 31.19483) |
22287 rows × 3 columns
In [5]:
gdf.hvplot(geo=True, tiles="OSM", frame_width=FSIZE, frame_height=FSIZE)
Out[5]:
In [6]:
tc = mpd.TrajectoryCollection(gdf, "ID", t="t")
In [7]:
evergiven = tc.get_trajectory(EVERID)
evergiven.hvplot(line_width=3, frame_width=FSIZE, frame_height=FSIZE)
Out[7]:
In [8]:
stop_detector = mpd.TrajectoryStopDetector(tc)
stop_pts = stop_detector.get_stop_points(
min_duration=timedelta(hours=3), max_diameter=1000
)
stop_pts
Out[8]:
geometry | start_time | end_time | traj_id | duration_s | |
---|---|---|---|---|---|
stop_id | |||||
2_2021-03-21 08:29:00 | POINT (32.35567 31.21248) | 2021-03-21 08:29:00 | 2021-03-21 23:11:00 | 2 | 52920.0 |
4_2021-03-23 22:23:00 | POINT (32.32796 31.39507) | 2021-03-23 22:23:00 | 2021-03-24 12:46:00 | 4 | 51780.0 |
5_2021-03-20 09:47:00 | POINT (32.35727 31.2179) | 2021-03-20 09:47:00 | 2021-03-21 03:55:00 | 5 | 65280.0 |
8_2021-03-23 06:15:00 | POINT (32.58077 30.00576) | 2021-03-23 06:15:00 | 2021-03-24 12:50:00 | 8 | 110100.0 |
9_2021-03-20 02:07:00 | POINT (32.43236 30.3034) | 2021-03-20 02:07:00 | 2021-03-20 05:56:00 | 9 | 13740.0 |
... | ... | ... | ... | ... | ... |
249_2021-03-20 06:30:00 | POINT (32.34406 30.36822) | 2021-03-20 06:30:00 | 2021-03-20 14:09:00 | 249 | 27540.0 |
250_2021-03-23 17:17:00 | POINT (32.53383 29.83297) | 2021-03-23 17:17:00 | 2021-03-24 12:21:00 | 250 | 68640.0 |
251_2021-03-23 22:21:00 | POINT (32.35181 31.45093) | 2021-03-23 22:21:00 | 2021-03-24 12:48:00 | 251 | 52020.0 |
255_2021-03-23 08:52:00 | POINT (32.57538 29.85072) | 2021-03-23 08:52:00 | 2021-03-24 11:14:00 | 255 | 94920.0 |
256_2021-03-20 00:25:00 | POINT (32.32908 31.19485) | 2021-03-20 00:25:00 | 2021-03-24 12:35:00 | 256 | 389400.0 |
258 rows × 5 columns
In [9]:
stop_pts["duration_h"] = stop_pts["duration_s"] / 3600
stop_pts
Out[9]:
geometry | start_time | end_time | traj_id | duration_s | duration_h | |
---|---|---|---|---|---|---|
stop_id | ||||||
2_2021-03-21 08:29:00 | POINT (32.35567 31.21248) | 2021-03-21 08:29:00 | 2021-03-21 23:11:00 | 2 | 52920.0 | 14.700000 |
4_2021-03-23 22:23:00 | POINT (32.32796 31.39507) | 2021-03-23 22:23:00 | 2021-03-24 12:46:00 | 4 | 51780.0 | 14.383333 |
5_2021-03-20 09:47:00 | POINT (32.35727 31.2179) | 2021-03-20 09:47:00 | 2021-03-21 03:55:00 | 5 | 65280.0 | 18.133333 |
8_2021-03-23 06:15:00 | POINT (32.58077 30.00576) | 2021-03-23 06:15:00 | 2021-03-24 12:50:00 | 8 | 110100.0 | 30.583333 |
9_2021-03-20 02:07:00 | POINT (32.43236 30.3034) | 2021-03-20 02:07:00 | 2021-03-20 05:56:00 | 9 | 13740.0 | 3.816667 |
... | ... | ... | ... | ... | ... | ... |
249_2021-03-20 06:30:00 | POINT (32.34406 30.36822) | 2021-03-20 06:30:00 | 2021-03-20 14:09:00 | 249 | 27540.0 | 7.650000 |
250_2021-03-23 17:17:00 | POINT (32.53383 29.83297) | 2021-03-23 17:17:00 | 2021-03-24 12:21:00 | 250 | 68640.0 | 19.066667 |
251_2021-03-23 22:21:00 | POINT (32.35181 31.45093) | 2021-03-23 22:21:00 | 2021-03-24 12:48:00 | 251 | 52020.0 | 14.450000 |
255_2021-03-23 08:52:00 | POINT (32.57538 29.85072) | 2021-03-23 08:52:00 | 2021-03-24 11:14:00 | 255 | 94920.0 | 26.366667 |
256_2021-03-20 00:25:00 | POINT (32.32908 31.19485) | 2021-03-20 00:25:00 | 2021-03-24 12:35:00 | 256 | 389400.0 | 108.166667 |
258 rows × 6 columns
Ever Given ran aground around 7:40 local time (5:40 UTC) on 23rd March 2021¶
In [10]:
stop_pts[stop_pts["traj_id"] == EVERID]
Out[10]:
geometry | start_time | end_time | traj_id | duration_s | duration_h | |
---|---|---|---|---|---|---|
stop_id | ||||||
235_2021-03-22 22:56:00 | POINT (32.55419 29.83488) | 2021-03-22 22:56:00 | 2021-03-23 02:14:00 | 235 | 11880.0 | 3.300000 |
235_2021-03-23 05:47:00 | POINT (32.58019 30.01763) | 2021-03-23 05:47:00 | 2021-03-24 12:52:00 | 235 | 111900.0 | 31.083333 |
In [11]:
map_plot = (
stop_pts.hvplot(geo=True, hover_cols=["start_time"], size=20, tiles="OSM")
* evergiven.hvplot(
line_width=5,
color="red",
frame_width=FSIZE,
frame_height=FSIZE,
alpha=0.5,
tiles=None,
).opts(active_tools=["pan", "wheelzoom"])
* stop_pts[stop_pts["traj_id"] == EVERID].hvplot(
geo=True,
hover_cols=["start_time"],
size=dim("duration_h") / 2,
color="red",
title="Trajectory & stop location of Ever Given and stops of other vessels",
)
)
scatter_plot = stop_pts.hvplot.scatter(
title="Stop start & duration (in hours)",
x="start_time",
y="duration_h",
frame_width=FSIZE,
frame_height=FSIZE,
alpha=0.7,
) * stop_pts[stop_pts["traj_id"] == EVERID].hvplot.scatter(
x="start_time", y="duration_h", color="red", size=200
)
map_plot + scatter_plot
Out[11]:
Data generously provided by VesselsValue.
In [12]:
stop_pts[stop_pts.start_time > datetime(2021, 3, 23, 5, 39, 0)].sort_values(
"duration_s", ascending=False
).head(
12
) # .style.background_gradient(cmap='Reds')
Out[12]:
geometry | start_time | end_time | traj_id | duration_s | duration_h | |
---|---|---|---|---|---|---|
stop_id | ||||||
235_2021-03-23 05:47:00 | POINT (32.58019 30.01763) | 2021-03-23 05:47:00 | 2021-03-24 12:52:00 | 235 | 111900.0 | 31.083333 |
8_2021-03-23 06:15:00 | POINT (32.58077 30.00576) | 2021-03-23 06:15:00 | 2021-03-24 12:50:00 | 8 | 110100.0 | 30.583333 |
168_2021-03-23 06:26:00 | POINT (32.58109 30.003) | 2021-03-23 06:26:00 | 2021-03-24 12:49:00 | 168 | 109380.0 | 30.383333 |
124_2021-03-23 06:53:00 | POINT (32.58854 29.77551) | 2021-03-23 06:53:00 | 2021-03-24 12:30:00 | 124 | 106620.0 | 29.616667 |
81_2021-03-23 07:48:00 | POINT (32.57137 29.83667) | 2021-03-23 07:48:00 | 2021-03-24 12:33:00 | 81 | 103500.0 | 28.750000 |
193_2021-03-23 08:04:00 | POINT (32.53971 29.92151) | 2021-03-23 08:04:00 | 2021-03-24 12:46:00 | 193 | 103320.0 | 28.700000 |
54_2021-03-23 08:48:00 | POINT (32.58387 29.98875) | 2021-03-23 08:48:00 | 2021-03-24 12:51:00 | 54 | 100980.0 | 28.050000 |
28_2021-03-23 09:38:00 | POINT (32.5705 29.85072) | 2021-03-23 09:38:00 | 2021-03-24 12:27:00 | 28 | 96540.0 | 26.816667 |
255_2021-03-23 08:52:00 | POINT (32.57538 29.85072) | 2021-03-23 08:52:00 | 2021-03-24 11:14:00 | 255 | 94920.0 | 26.366667 |
207_2021-03-23 10:58:00 | POINT (32.3886 30.32303) | 2021-03-23 10:58:00 | 2021-03-24 12:35:00 | 207 | 92220.0 | 25.616667 |
82_2021-03-23 11:04:00 | POINT (32.41836 30.29288) | 2021-03-23 11:04:00 | 2021-03-24 12:40:00 | 82 | 92160.0 | 25.600000 |
115_2021-03-23 11:56:00 | POINT (32.40123 30.34127) | 2021-03-23 11:56:00 | 2021-03-24 12:17:00 | 115 | 87660.0 | 24.350000 |