Detecting stops¶
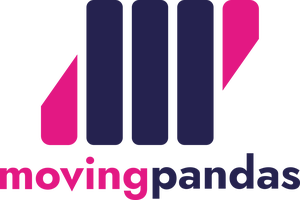
There are no definitive answers when it comes to detecting / extracting stops from movement trajectories. Due to tracking inaccuracies, movement speed rarely goes to true zero. GPS tracks, for example, tend to keep moving around the object's stop location.
Suitable stop definitions are also highly application dependent. For example, an application may be interested in analyzing trip purposes. To do so, analysts would be interested in stops that are longer than, for example, 5 minutes and may try to infer the purpose of the stop from the stop location and time. Shorter stops, such as delays at traffic lights, however would not be relevant for this appication.
In the MovingPandas TrajectoryStopDetector implementation, a stop is detected if the movement stays within an area of specified size for at least the specified duration.
import pandas as pd
import geopandas as gpd
import movingpandas as mpd
import shapely as shp
import hvplot.pandas
import matplotlib.pyplot as plt
import folium
from geopandas import GeoDataFrame, read_file
from shapely.geometry import Point, LineString, Polygon
from datetime import datetime, timedelta
from holoviews import opts
import warnings
warnings.filterwarnings("ignore")
plot_defaults = {"linewidth": 5, "capstyle": "round", "figsize": (9, 3), "legend": True}
opts.defaults(
opts.Overlay(active_tools=["wheel_zoom"], frame_width=500, frame_height=400)
)
mpd.show_versions()
MovingPandas 0.20.0 SYSTEM INFO ----------- python : 3.10.15 | packaged by conda-forge | (main, Oct 16 2024, 01:15:49) [MSC v.1941 64 bit (AMD64)] executable : c:\Users\Agarkovam\AppData\Local\miniforge3\envs\mpd-ex\python.exe machine : Windows-10-10.0.19045-SP0 GEOS, GDAL, PROJ INFO --------------------- GEOS : None GEOS lib : None GDAL : None GDAL data dir: None PROJ : 9.5.0 PROJ data dir: C:\Users\Agarkovam\AppData\Local\miniforge3\envs\mpd-ex\Library\share\proj PYTHON DEPENDENCIES ------------------- geopandas : 1.0.1 pandas : 2.2.3 fiona : None numpy : 1.23.1 shapely : 2.0.6 pyproj : 3.7.0 matplotlib : 3.9.2 mapclassify: 2.8.1 geopy : 2.4.1 holoviews : 1.20.0 hvplot : 0.11.1 geoviews : 1.13.0 stonesoup : 1.4
gdf = read_file("../data/geolife_small.gpkg")
tc = mpd.TrajectoryCollection(gdf, "trajectory_id", t="t")
Stop detection with a single Trajectory¶
my_traj = tc.trajectories[0]
my_traj
Trajectory 1 (2008-12-11 04:42:14 to 2008-12-11 05:15:46) | Size: 466 | Length: 6207.0m Bounds: (116.385602, 39.862378, 116.393553, 39.898723) LINESTRING (116.391305 39.898573, 116.391317 39.898617, 116.390928 39.898613, 116.390833 39.898635,
traj_plot = my_traj.hvplot(
title="Trajectory {}".format(my_traj.id),
line_width=7.0,
tiles="CartoLight",
color="slategray",
)
traj_plot
detector = mpd.TrajectoryStopDetector(my_traj)
Stop duration¶
%%time
stop_time_ranges = detector.get_stop_time_ranges(
min_duration=timedelta(seconds=60), max_diameter=100
)
CPU times: total: 266 ms Wall time: 296 ms
for x in stop_time_ranges:
print(x)
Traj 1: 2008-12-11 04:42:14 - 2008-12-11 04:43:32 (duration: 0 days 00:01:18) Traj 1: 2008-12-11 04:43:52 - 2008-12-11 04:47:40 (duration: 0 days 00:03:48) Traj 1: 2008-12-11 04:50:06 - 2008-12-11 04:51:23 (duration: 0 days 00:01:17) Traj 1: 2008-12-11 04:54:50 - 2008-12-11 04:55:55 (duration: 0 days 00:01:05) Traj 1: 2008-12-11 05:02:03 - 2008-12-11 05:06:34 (duration: 0 days 00:04:31) Traj 1: 2008-12-11 05:07:19 - 2008-12-11 05:08:31 (duration: 0 days 00:01:12) Traj 1: 2008-12-11 05:11:17 - 2008-12-11 05:13:38 (duration: 0 days 00:02:21) Traj 1: 2008-12-11 05:13:51 - 2008-12-11 05:15:46 (duration: 0 days 00:01:55)
Stop points¶
%%time
stop_points = detector.get_stop_points(
min_duration=timedelta(seconds=60), max_diameter=100
)
CPU times: total: 234 ms Wall time: 232 ms
stop_points
geometry | start_time | end_time | traj_id | duration_s | |
---|---|---|---|---|---|
stop_id | |||||
1_2008-12-11 04:42:14 | POINT (116.39112 39.89862) | 2008-12-11 04:42:14 | 2008-12-11 04:43:32 | 1 | 78.0 |
1_2008-12-11 04:43:52 | POINT (116.3907 39.89838) | 2008-12-11 04:43:52 | 2008-12-11 04:47:40 | 1 | 228.0 |
1_2008-12-11 04:50:06 | POINT (116.38932 39.88923) | 2008-12-11 04:50:06 | 2008-12-11 04:51:23 | 1 | 77.0 |
1_2008-12-11 04:54:50 | POINT (116.39232 39.87849) | 2008-12-11 04:54:50 | 2008-12-11 04:55:55 | 1 | 65.0 |
1_2008-12-11 05:02:03 | POINT (116.39293 39.86384) | 2008-12-11 05:02:03 | 2008-12-11 05:06:34 | 1 | 271.0 |
1_2008-12-11 05:07:19 | POINT (116.39024 39.86383) | 2008-12-11 05:07:19 | 2008-12-11 05:08:31 | 1 | 72.0 |
1_2008-12-11 05:11:17 | POINT (116.38596 39.86519) | 2008-12-11 05:11:17 | 2008-12-11 05:13:38 | 1 | 141.0 |
1_2008-12-11 05:13:51 | POINT (116.38603 39.86538) | 2008-12-11 05:13:51 | 2008-12-11 05:15:46 | 1 | 115.0 |
stop_point_plot = traj_plot * stop_points.hvplot(
geo=True, size="duration_s", color="deeppink"
)
stop_point_plot
stop_points_gdf = gpd.GeoDataFrame(stop_points, geometry="geometry", crs="EPSG:4326")
m = my_traj.explore(
color="blue",
style_kwds={"weight": 4},
name="Trajectory",
)
stop_points_gdf.explore(
m=m,
color="red",
style_kwds={
"style_function": lambda x: {"radius": x["properties"]["duration_s"] / 10}
},
name="Stop points",
)
folium.TileLayer("OpenStreetMap").add_to(m)
folium.LayerControl().add_to(m)
m
Stop segments¶
%%time
stop_segments = detector.get_stop_segments(
min_duration=timedelta(seconds=60), max_diameter=100
)
CPU times: total: 219 ms Wall time: 219 ms
stop_segments
TrajectoryCollection with 8 trajectories
stop_segment_plot = stop_point_plot * stop_segments.hvplot(
line_width=7.0, tiles=None, color="orange"
)
stop_segment_plot
m = my_traj.explore(
color="blue",
tooltip="trajectory_id",
popup=True,
style_kwds={"weight": 4},
name="Trajectory",
)
stop_segments.explore(
m=m,
color="orange",
tooltip="trajectory_id",
popup=True,
style_kwds={"weight": 4},
name="Stop segments",
)
stop_points_gdf.explore(
m=m,
color="red",
tooltip="stop_id",
popup=True,
marker_kwds={"radius": 3},
name="Stop points",
)
folium.TileLayer("CartoDB positron").add_to(m)
folium.LayerControl().add_to(m)
m
Split at stops¶
%%time
split = mpd.StopSplitter(my_traj).split(
min_duration=timedelta(seconds=60), max_diameter=100
)
CPU times: total: 250 ms Wall time: 250 ms
split
TrajectoryCollection with 7 trajectories
split.to_traj_gdf()
trajectory_id | start_t | end_t | geometry | length | direction | |
---|---|---|---|---|---|---|
0 | 1_2008-12-11 04:43:32 | 2008-12-11 04:43:32 | 2008-12-11 04:43:52 | LINESTRING (116.39083 39.89864, 116.38941 39.8... | 264.335588 | 192.205758 |
1 | 1_2008-12-11 04:47:40 | 2008-12-11 04:47:40 | 2008-12-11 04:50:06 | LINESTRING (116.39019 39.89844, 116.39013 39.8... | 1070.704027 | 185.938120 |
2 | 1_2008-12-11 04:51:23 | 2008-12-11 04:51:23 | 2008-12-11 04:54:50 | LINESTRING (116.39 39.88924, 116.3901 39.88926... | 1378.618493 | 169.324962 |
3 | 1_2008-12-11 04:55:55 | 2008-12-11 04:55:55 | 2008-12-11 05:02:03 | LINESTRING (116.39183 39.87814, 116.39174 39.8... | 1909.066931 | 176.111541 |
4 | 1_2008-12-11 05:06:34 | 2008-12-11 05:06:34 | 2008-12-11 05:07:19 | LINESTRING (116.39251 39.86398, 116.39239 39.8... | 145.517971 | 262.952795 |
5 | 1_2008-12-11 05:08:31 | 2008-12-11 05:08:31 | 2008-12-11 05:11:17 | LINESTRING (116.38974 39.86382, 116.38967 39.8... | 439.686682 | 300.319621 |
6 | 1_2008-12-11 05:13:38 | 2008-12-11 05:13:38 | 2008-12-11 05:13:51 | LINESTRING (116.38587 39.86544, 116.38584 39.8... | 16.921893 | 349.846256 |
split.explore(
column="trajectory_id", tiles="CartoDB dark_matter", style_kwds={"weight": 4}
)
stop_segment_plot + split.hvplot(
title="Trajectory {} split at stops".format(my_traj.id),
line_width=7.0,
tiles="CartoLight",
)
Stop Detection for TrajectoryCollections¶
The process is the same as for individual trajectories.
%%time
detector = mpd.TrajectoryStopDetector(tc)
stop_points = detector.get_stop_points(
min_duration=timedelta(seconds=120), max_diameter=100
)
len(stop_points)
CPU times: total: 3.02 s Wall time: 3.06 s
31
ax = tc.plot(figsize=(7, 7))
stop_points.plot(ax=ax, color="red")
<Axes: >
m = tc.explore(
column="trajectory_id",
tooltip="trajectory_id",
popup=True,
style_kwds={"weight": 4},
name="Trajectories",
)
stop_points.explore(
m=m,
color="red",
tooltip="stop_id",
popup=True,
marker_kwds={"radius": 5},
name="Stop points",
)
folium.TileLayer("CartoDB positron").add_to(m)
folium.LayerControl().add_to(m)
m