Exporting Trajectories¶
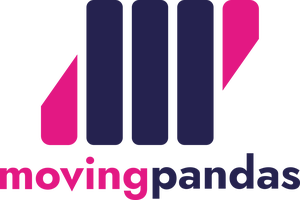
Trajectories and TrajectoryCollections can be converted back to GeoDataFrames that can then be exported to different GIS file formats.
In [1]:
import pandas as pd
import geopandas as gpd
import movingpandas as mpd
import shapely as shp
import hvplot.pandas
from geopandas import GeoDataFrame, read_file
from shapely.geometry import Point, LineString, Polygon
from datetime import datetime, timedelta
from holoviews import opts
import warnings
warnings.filterwarnings("ignore")
opts.defaults(
opts.Overlay(active_tools=["wheel_zoom"], frame_width=500, frame_height=400)
)
mpd.show_versions()
MovingPandas 0.20.0 SYSTEM INFO ----------- python : 3.10.15 | packaged by conda-forge | (main, Oct 16 2024, 01:15:49) [MSC v.1941 64 bit (AMD64)] executable : c:\Users\Agarkovam\AppData\Local\miniforge3\envs\mpd-ex\python.exe machine : Windows-10-10.0.19045-SP0 GEOS, GDAL, PROJ INFO --------------------- GEOS : None GEOS lib : None GDAL : None GDAL data dir: None PROJ : 9.5.0 PROJ data dir: C:\Users\Agarkovam\AppData\Local\miniforge3\envs\mpd-ex\Library\share\proj PYTHON DEPENDENCIES ------------------- geopandas : 1.0.1 pandas : 2.2.3 fiona : None numpy : 1.23.1 shapely : 2.0.6 pyproj : 3.7.0 matplotlib : 3.9.2 mapclassify: 2.8.1 geopy : 2.4.1 holoviews : 1.20.0 hvplot : 0.11.1 geoviews : 1.13.0 stonesoup : 1.4
In [2]:
gdf = read_file("../data/geolife_small.gpkg")
tc = mpd.TrajectoryCollection(gdf, "trajectory_id", t="t")
tc
Out[2]:
TrajectoryCollection with 5 trajectories
In [3]:
tc.to_point_gdf()
Out[3]:
id | sequence | trajectory_id | tracker | geometry | |
---|---|---|---|---|---|
t | |||||
2008-12-11 04:42:14 | 1 | 1 | 1 | 19 | POINT (116.3913 39.89857) |
2008-12-11 04:42:16 | 2 | 2 | 1 | 19 | POINT (116.39132 39.89862) |
2008-12-11 04:43:26 | 3 | 3 | 1 | 19 | POINT (116.39093 39.89861) |
2008-12-11 04:43:32 | 4 | 4 | 1 | 19 | POINT (116.39083 39.89864) |
2008-12-11 04:43:47 | 5 | 5 | 1 | 19 | POINT (116.38941 39.89872) |
... | ... | ... | ... | ... | ... |
2009-02-25 14:31:04 | 6993 | 867 | 5 | 2 | POINT (116.33719 39.92623) |
2009-02-25 14:31:09 | 6994 | 868 | 5 | 2 | POINT (116.33721 39.92624) |
2009-02-25 14:31:14 | 6995 | 869 | 5 | 2 | POINT (116.33726 39.92621) |
2009-02-25 14:31:19 | 6996 | 870 | 5 | 2 | POINT (116.33729 39.9262) |
2009-02-25 14:31:24 | 6997 | 871 | 5 | 2 | POINT (116.33733 39.92619) |
5908 rows × 5 columns
Convert to a line GeoDataFrame¶
In [4]:
tc.to_line_gdf()
Out[4]:
id | sequence | trajectory_id | tracker | t | prev_t | geometry | |
---|---|---|---|---|---|---|---|
0 | 2 | 2 | 1 | 19 | 2008-12-11 04:42:16 | 2008-12-11 04:42:14 | LINESTRING (116.3913 39.89857, 116.39132 39.89... |
1 | 3 | 3 | 1 | 19 | 2008-12-11 04:43:26 | 2008-12-11 04:42:16 | LINESTRING (116.39132 39.89862, 116.39093 39.8... |
2 | 4 | 4 | 1 | 19 | 2008-12-11 04:43:32 | 2008-12-11 04:43:26 | LINESTRING (116.39093 39.89861, 116.39083 39.8... |
3 | 5 | 5 | 1 | 19 | 2008-12-11 04:43:47 | 2008-12-11 04:43:32 | LINESTRING (116.39083 39.89864, 116.38941 39.8... |
4 | 6 | 6 | 1 | 19 | 2008-12-11 04:43:50 | 2008-12-11 04:43:47 | LINESTRING (116.38941 39.89872, 116.39052 39.8... |
... | ... | ... | ... | ... | ... | ... | ... |
5898 | 6993 | 867 | 5 | 2 | 2009-02-25 14:31:04 | 2009-02-25 14:31:02 | LINESTRING (116.33715 39.92631, 116.33719 39.9... |
5899 | 6994 | 868 | 5 | 2 | 2009-02-25 14:31:09 | 2009-02-25 14:31:04 | LINESTRING (116.33719 39.92623, 116.33721 39.9... |
5900 | 6995 | 869 | 5 | 2 | 2009-02-25 14:31:14 | 2009-02-25 14:31:09 | LINESTRING (116.33721 39.92624, 116.33726 39.9... |
5901 | 6996 | 870 | 5 | 2 | 2009-02-25 14:31:19 | 2009-02-25 14:31:14 | LINESTRING (116.33726 39.92621, 116.33729 39.9... |
5902 | 6997 | 871 | 5 | 2 | 2009-02-25 14:31:24 | 2009-02-25 14:31:19 | LINESTRING (116.33729 39.9262, 116.33733 39.92... |
5903 rows × 7 columns
Convert to a trajectory GeoDataFrame¶
In [5]:
tc.to_traj_gdf(wkt=True)
Out[5]:
trajectory_id | start_t | end_t | geometry | length | direction | wkt | |
---|---|---|---|---|---|---|---|
0 | 1 | 2008-12-11 04:42:14 | 2008-12-11 05:15:46 | LINESTRING (116.3913 39.89857, 116.39132 39.89... | 6207.020261 | 186.681376 | LINESTRING M (116.391305 39.898573 1228970534.... |
1 | 2 | 2009-06-29 07:02:25 | 2009-06-29 11:13:12 | LINESTRING (116.59096 40.07196, 116.5909 40.07... | 38764.575483 | 250.585295 | LINESTRING M (116.590957 40.071961 1246258945.... |
2 | 3 | 2009-02-04 04:32:53 | 2009-02-04 11:20:12 | LINESTRING (116.38569 39.89977, 116.38565 39.8... | 12745.157506 | 304.115160 | LINESTRING M (116.385689 39.899773 1233721973.... |
3 | 4 | 2009-03-10 10:36:45 | 2009-03-10 12:01:07 | LINESTRING (116.38805 39.90342, 116.38804 39.9... | 14363.780551 | 300.732843 | LINESTRING M (116.388053 39.903418 1236681405.... |
4 | 5 | 2009-02-25 09:47:03 | 2009-02-25 14:31:24 | LINESTRING (116.38526 39.90027, 116.38525 39.9... | 39259.779560 | 305.200501 | LINESTRING M (116.385256 39.90027 1235555223.0... |
In [6]:
tc.to_traj_gdf(agg={"tracker": "mode", "sequence": ["min", "max"]})
Out[6]:
trajectory_id | start_t | end_t | geometry | length | direction | tracker_mode | sequence_min | sequence_max | |
---|---|---|---|---|---|---|---|---|---|
0 | 1 | 2008-12-11 04:42:14 | 2008-12-11 05:15:46 | LINESTRING (116.3913 39.89857, 116.39132 39.89... | 6207.020261 | 186.681376 | 19 | 1 | 466 |
1 | 2 | 2009-06-29 07:02:25 | 2009-06-29 11:13:12 | LINESTRING (116.59096 40.07196, 116.5909 40.07... | 38764.575483 | 250.585295 | 0 | 1090 | 1986 |
2 | 3 | 2009-02-04 04:32:53 | 2009-02-04 11:20:12 | LINESTRING (116.38569 39.89977, 116.38565 39.8... | 12745.157506 | 304.115160 | 2 | 1 | 1810 |
3 | 4 | 2009-03-10 10:36:45 | 2009-03-10 12:01:07 | LINESTRING (116.38805 39.90342, 116.38804 39.9... | 14363.780551 | 300.732843 | 2 | 1 | 1864 |
4 | 5 | 2009-02-25 09:47:03 | 2009-02-25 14:31:24 | LINESTRING (116.38526 39.90027, 116.38525 39.9... | 39259.779560 | 305.200501 | 2 | 1 | 871 |
Exporting to GIS file formats¶
These GeoDataFrames can be exported to different file formats using GeoPandas, as documented in https://geopandas.org/docs/user_guide/io.html
In [7]:
export_gdf = tc.to_traj_gdf(agg={"sequence": ["min", "max"]})
export_gdf.to_file("temp.gpkg", layer="trajectories", driver="GPKG")
In [8]:
read_file("temp.gpkg").plot()
Out[8]:
<Axes: >
In [9]:
read_file("temp.gpkg")
Out[9]:
trajectory_id | start_t | end_t | length | direction | sequence_min | sequence_max | geometry | |
---|---|---|---|---|---|---|---|---|
0 | 1 | 2008-12-11 04:42:14 | 2008-12-11 05:15:46 | 6207.020261 | 186.681376 | 1 | 466 | LINESTRING (116.3913 39.89857, 116.39132 39.89... |
1 | 2 | 2009-06-29 07:02:25 | 2009-06-29 11:13:12 | 38764.575483 | 250.585295 | 1090 | 1986 | LINESTRING (116.59096 40.07196, 116.5909 40.07... |
2 | 3 | 2009-02-04 04:32:53 | 2009-02-04 11:20:12 | 12745.157506 | 304.115160 | 1 | 1810 | LINESTRING (116.38569 39.89977, 116.38565 39.8... |
3 | 4 | 2009-03-10 10:36:45 | 2009-03-10 12:01:07 | 14363.780551 | 300.732843 | 1 | 1864 | LINESTRING (116.38805 39.90342, 116.38804 39.9... |
4 | 5 | 2009-02-25 09:47:03 | 2009-02-25 14:31:24 | 39259.779560 | 305.200501 | 1 | 871 | LINESTRING (116.38526 39.90027, 116.38525 39.9... |
In [ ]: