Extracting mover positions¶
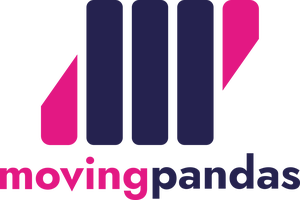
The following examples show how to find a mover's position at a certain time or for a certain time span.
In [1]:
import pandas as pd
import geopandas as gpd
import movingpandas as mpd
import shapely as shp
import hvplot.pandas
from geopandas import GeoDataFrame, read_file
from shapely.geometry import Point, LineString, Polygon
from datetime import datetime, timedelta
from holoviews import opts
import warnings
warnings.filterwarnings("ignore")
opts.defaults(
opts.Overlay(active_tools=["wheel_zoom"], frame_width=500, frame_height=400)
)
mpd.show_versions()
MovingPandas 0.20.0 SYSTEM INFO ----------- python : 3.10.15 | packaged by conda-forge | (main, Oct 16 2024, 01:15:49) [MSC v.1941 64 bit (AMD64)] executable : c:\Users\Agarkovam\AppData\Local\miniforge3\envs\mpd-ex\python.exe machine : Windows-10-10.0.19045-SP0 GEOS, GDAL, PROJ INFO --------------------- GEOS : None GEOS lib : None GDAL : None GDAL data dir: None PROJ : 9.5.0 PROJ data dir: C:\Users\Agarkovam\AppData\Local\miniforge3\envs\mpd-ex\Library\share\proj PYTHON DEPENDENCIES ------------------- geopandas : 1.0.1 pandas : 2.2.3 fiona : None numpy : 1.23.1 shapely : 2.0.6 pyproj : 3.7.0 matplotlib : 3.9.2 mapclassify: 2.8.1 geopy : 2.4.1 holoviews : 1.20.0 hvplot : 0.11.1 geoviews : 1.13.0 stonesoup : 1.4
First, let's create a basic trajectory:
In [2]:
df = pd.DataFrame(
[
{"geometry": Point(0, 0), "t": datetime(2018, 1, 1, 12, 0, 0)},
{"geometry": Point(6, 0), "t": datetime(2018, 1, 1, 12, 6, 0)},
{"geometry": Point(6, 6), "t": datetime(2018, 1, 1, 12, 10, 0)},
{"geometry": Point(9, 9), "t": datetime(2018, 1, 1, 12, 15, 0)},
]
).set_index("t")
gdf = GeoDataFrame(df, crs=31256)
toy_traj = mpd.Trajectory(gdf, 1)
toy_traj
Out[2]:
Trajectory 1 (2018-01-01 12:00:00 to 2018-01-01 12:15:00) | Size: 4 | Length: 16.2m Bounds: (0.0, 0.0, 9.0, 9.0) LINESTRING (0 0, 6 0, 6 6, 9 9)
In [3]:
ax = toy_traj.plot()
gpd.GeoSeries(toy_traj.get_start_location()).plot(ax=ax, color="blue")
gpd.GeoSeries(toy_traj.get_end_location()).plot(ax=ax, color="red")
Out[3]:
<Axes: >
Extracting a mover's position at a certain time¶
When we call this method, the resulting point is directly rendered:
In [4]:
toy_traj.get_position_at(datetime(2018, 1, 1, 12, 6, 0), method="nearest")
Out[4]:
To see its coordinates, we can look at the print output:
In [5]:
print(toy_traj.get_position_at(datetime(2018, 1, 1, 12, 6, 0), method="nearest"))
POINT (6 0)
The method parameter describes what the function should do if there is no entry in the trajectory GeoDataFrame for the specified timestamp.
For example, there is no entry at 2018-01-01 12:07:00
In [6]:
toy_traj.df
Out[6]:
geometry | traj_id | |
---|---|---|
t | ||
2018-01-01 12:00:00 | POINT (0 0) | 1 |
2018-01-01 12:06:00 | POINT (6 0) | 1 |
2018-01-01 12:10:00 | POINT (6 6) | 1 |
2018-01-01 12:15:00 | POINT (9 9) | 1 |
In [7]:
t = datetime(2018, 1, 1, 12, 7, 0)
print(toy_traj.get_position_at(t, method="nearest"))
print(toy_traj.get_position_at(t, method="interpolated"))
print(toy_traj.get_position_at(t, method="ffill")) # from the previous row
print(toy_traj.get_position_at(t, method="bfill")) # from the following row
POINT (6 0) POINT (6 1.5) POINT (6 0) POINT (6 6)
In [8]:
point = toy_traj.get_position_at(t, method="interpolated")
ax = toy_traj.plot()
gpd.GeoSeries(point).plot(ax=ax, color="red", markersize=100)
Out[8]:
<Axes: >
Extracting trajectory segments based on time¶
First, let's extract the trajectory segment for a certain time period:
In [9]:
segment = toy_traj.get_segment_between(
datetime(2018, 1, 1, 12, 6, 0), datetime(2018, 1, 1, 12, 12, 0)
)
print(segment)
Trajectory 1_2018-01-01 12:06:00 (2018-01-01 12:06:00 to 2018-01-01 12:10:00) | Size: 2 | Length: 6.0m Bounds: (6.0, 0.0, 6.0, 6.0) LINESTRING (6 0, 6 6)
In [10]:
ax = toy_traj.plot()
segment.plot(ax=ax, color="red", linewidth=5)
Out[10]:
<Axes: >
Extracting trajectory segments based on geometry (i.e. clipping)¶
Now, let's extract the trajectory segment that intersects with a given polygon:
In [11]:
xmin, xmax, ymin, ymax = 2, 8, -10, 5
polygon = Polygon(
[(xmin, ymin), (xmin, ymax), (xmax, ymax), (xmax, ymin), (xmin, ymin)]
)
polygon
Out[11]:
In [12]:
intersections = toy_traj.clip(polygon)
intersections
Out[12]:
TrajectoryCollection with 1 trajectories
In [13]:
ax = toy_traj.plot()
gpd.GeoSeries(polygon).plot(ax=ax, color="lightgray")
intersections.plot(ax=ax, color="red", linewidth=5, capstyle="round")
Out[13]:
<Axes: >
In [ ]: