Measuring distances¶
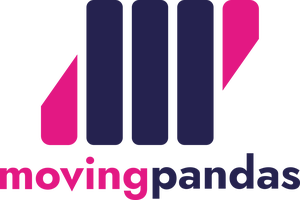
Distances can be computed between trajectories as well as between trajectories and other geometry objects. The implemented distance measures are:
In [1]:
import pandas as pd
import geopandas as gpd
import movingpandas as mpd
import shapely as shp
import hvplot.pandas
import matplotlib.pyplot as plt
from geopandas import GeoDataFrame, read_file
from shapely.geometry import Point, LineString, Polygon
from datetime import datetime, timedelta
from holoviews import opts, dim
import warnings
warnings.filterwarnings('ignore')
plot_defaults = {'linewidth':5, 'capstyle':'round', 'figsize':(9,3), 'legend':True}
opts.defaults(opts.Overlay(active_tools=['wheel_zoom']))
hvplot_defaults = {'tiles':'CartoLight', 'frame_height':320, 'frame_width':320, 'cmap':'Viridis', 'colorbar':True}
mpd.show_versions()
MovingPandas 0.17.0 SYSTEM INFO ----------- python : 3.10.12 | packaged by conda-forge | (main, Jun 23 2023, 22:34:57) [MSC v.1936 64 bit (AMD64)] executable : H:\miniconda3\envs\mpd-ex\python.exe machine : Windows-10-10.0.19045-SP0 GEOS, GDAL, PROJ INFO --------------------- GEOS : None GEOS lib : None GDAL : 3.7.0 GDAL data dir: None PROJ : 9.2.1 PROJ data dir: H:\miniconda3\pkgs\proj-9.0.0-h1cfcee9_1\Library\share\proj PYTHON DEPENDENCIES ------------------- geopandas : 0.13.2 pandas : 2.0.3 fiona : 1.9.4 numpy : 1.24.4 shapely : 2.0.1 rtree : 1.0.1 pyproj : 3.6.0 matplotlib : 3.7.2 mapclassify: 2.5.0 geopy : 2.3.0 holoviews : 1.17.0 hvplot : 0.8.3 geoviews : 1.9.6 stonesoup : 1.0
Measuring distances between trajectories¶
In [2]:
df = pd.DataFrame([
{'geometry':Point(0,0), 't':datetime(2018,1,1,12,0,0)},
{'geometry':Point(6,0), 't':datetime(2018,1,1,12,6,0)},
{'geometry':Point(6,6), 't':datetime(2018,1,1,12,10,0)},
{'geometry':Point(9,9), 't':datetime(2018,1,1,12,15,0)}
]).set_index('t')
geo_df = GeoDataFrame(df, crs=31256)
toy_traj = mpd.Trajectory(geo_df, 1)
toy_traj.df
Out[2]:
geometry | traj_id | |
---|---|---|
t | ||
2018-01-01 12:00:00 | POINT (0.000 0.000) | 1 |
2018-01-01 12:06:00 | POINT (6.000 0.000) | 1 |
2018-01-01 12:10:00 | POINT (6.000 6.000) | 1 |
2018-01-01 12:15:00 | POINT (9.000 9.000) | 1 |
In [3]:
df = pd.DataFrame([
{'geometry':Point(3,3), 't':datetime(2018,1,1,12,0,0)},
{'geometry':Point(3,9), 't':datetime(2018,1,1,12,6,0)},
{'geometry':Point(2,9), 't':datetime(2018,1,1,12,10,0)},
{'geometry':Point(0,7), 't':datetime(2018,1,1,12,15,0)}
]).set_index('t')
geo_df = GeoDataFrame(df, crs=31256)
toy_traj2 = mpd.Trajectory(geo_df, 1)
toy_traj2.df
ax = toy_traj.plot()
toy_traj2.plot(ax=ax, color='red')
Out[3]:
<Axes: >
In [4]:
print(f'Distance: {toy_traj.distance(toy_traj2)} meters')
print(f'Hausdorff distance: {toy_traj.hausdorff_distance(toy_traj2):.2f} meters')
Distance: 3.0 meters Hausdorff distance: 6.08 meters
In [5]:
print(f'Distance: {toy_traj.distance(toy_traj2, units="cm")} cm')
print(f'Hausdorff distance: {toy_traj.hausdorff_distance(toy_traj2, units="km"):.6f} km')
Distance: 300.0 cm Hausdorff distance: 0.006083 km
Measuring distances between trajectories and other geometry objects¶
In [6]:
pt = Point(1, 5)
line = LineString([(3,3), (3,9)])
ax = toy_traj.plot()
gpd.GeoSeries(pt).plot(ax=ax, color='red')
gpd.GeoSeries(line).plot(ax=ax, color='red')
Out[6]:
<Axes: >
In [7]:
print(f'Distance: {toy_traj.distance(pt)}')
print(f'Hausdorff distance: {toy_traj.hausdorff_distance(pt):.2f}')
Distance: 5.0 Hausdorff distance: 8.94
In [8]:
print(f'Distance: {toy_traj.distance(line)}')
print(f'Hausdorff distance: {toy_traj.hausdorff_distance(line)}')
Distance: 3.0 Hausdorff distance: 6.0
In [9]:
print(f'Distance: {toy_traj.distance(line, units="cm")} cm')
print(f'Hausdorff distance: {toy_traj.hausdorff_distance(line, units="km"):.6f} km')
Distance: 300.0 cm Hausdorff distance: 0.006000 km
In [ ]: